How to create hide/show FAQs in Squarespace
More in Plugins, Scripting and Styling
Here's a concise solution for creating hide/show text in Squarespace pages, using a Markdown Block and a jQuery script.
The code on this page is free for you to install on your Squarespace site.
+ How does it work?
The solution uses a Markdown block to hold the questions and answers. For this version of the script it should be the only Markdown block on the page, though I may improve the script at a later date to allow for multiple open/close areas.
A jQuery script then manipulates the Markdown block to add the open/close behaviour.
+ Why Markdown?
The native Squarespace html layouts can be difficult to interpret. Placing a single Markdown block on a page makes it very easy to target with scripting as it has a class of .markdown-block
.
Markdown is also easier for a non technical user to edit or add content compared to raw html.
- It supports bullet points and other styling codes
Markdown blocks can also include html, which opens up the possibility for richer experiences that include imagery and other embedded media.
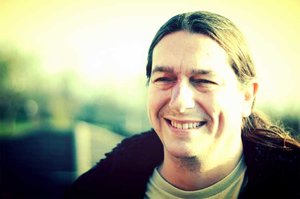
+ Why jQuery
jQuery is a Javascript framework that removes a lot of complexity when coding solutions that need to work on multiple web browsers.
This open/close functionality is expressed in 4 lines of jQuery code (excluding the call to the main jQuery library) whereas a pure Javascript solution would be far longer.
It's true that the jQuery library can add page load time, but in my code I link to the one hosted on Google's content delivery network, which means there's a strong chance that a user already has the jQuery library in their browser cache.
The solution
NB. Code samples may be reused subject to certain conditions.
The Markdown
IMPORTANT: The content of the FAQ Questions & Answers MUST be inserted into a Markdown Block. Using a standard text block or code block won't work.
Here's the Markdown code that drives the example at the top of this page. I've edited out a lot of the content to make is easier to read.
## + How does it work? The solution uses a Markdown block... ## + Why Markdown? The native Squarespace.. * It supports bullet points and *other* **styling** _codes_ Markdown blocks can also include html... <img src="http://static1.squarespace.com/static/51027975e4b014593379c8b3/t/512f891fe4b0c3388795e1ce/1362070062224/headshot2.jpg?format=200w" /> ## + Why jQuery jQuery is a...
## Denotes an H2 heading. The script interprets these as the links that open/close the content sections.
The + sign is used as a signifier that the heading can be opened.
The jQuery code
You need the following code call in either your sitewide code injection point or on the page's code injection point. If you already using jQuery scripts elsewhere on your site you can omit this line.
<script type="text/javascript" src="//ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js"></script>
Next up we have the code that does all of the work.
First off there's a clean version for copying and pasting into your page's code injection point. After that I've included an annotated version so that you can understand what's going on.
Clean version:
<script> $(document).ready(function(){ $('.markdown-block .sqs-block-content h2').css('cursor','pointer'); $(".markdown-block .sqs-block-content h2").nextUntil("h2").slideToggle(); $(".markdown-block .sqs-block-content h2").click(function() {$(this).nextUntil("h2").slideToggle();}); }); </script>
Annotated version:
<script> // Document ready tells the script to fire only once the entire page is loaded. $(document).ready(function(){ // The next line tells the mouse cursor to becom a pointer when hovering over the H2 elements in the Markdown. $('.markdown-block .sqs-block-content h2').css('cursor','pointer'); // The next line initialises the open/close areas by hiding the content $(".markdown-block .sqs-block-content h2").nextUntil("h2").slideToggle(); //Finally, there is a function that listens for a click on an H2 and either shows or hides its content depending on its current state. $(".markdown-block .sqs-block-content h2").click(function() {$(this).nextUntil("h2").slideToggle();}); }); </script>
Variation 1 - Making displayed answer retract when a new FAQ is clicked:
<script> $(document).ready(function(){ $('.markdown-block .sqs-block-content h2').css('cursor','pointer'); $(".markdown-block .sqs-block-content h2").nextUntil("h2").slideToggle(); $(".markdown-block .sqs-block-content h2").click(function() { $(".markdown-block .sqs-block-content h2").nextUntil("h2").slideUp(); $(this).nextUntil("h2").slideDown(); }); }); </script>
Variation 2 - Toggling the + / - symbols
First, the script:
<script> $(document).ready(function(){ $('.markdown-block .sqs-block-content h2').addClass('ui-closed').css('cursor','pointer'); $(".markdown-block .sqs-block-content h2").nextUntil("h2").slideToggle(); $(".markdown-block .sqs-block-content h2").click(function() { $(this).nextUntil("h2").slideToggle(); $(this).toggleClass('ui-closed ui-open'); }); }); </script>
Then some custom css
.markdown-block p { margin-left:1.5em; } .markdown-block .ui-closed:before { font-family:monospace; content:"+ "; } .markdown-block .ui-open:before { font-family:monospace; content:"- "; }
Thank you so much, you saved my life!!
Hello,
Thank you very much for sharing the above code. When i use any of the above versions, the page loads half of the content on it. Just wondering if there is a workaround.
Hi, I've been using your code for users to have optional access to transcripts and it looks and works great, thank you! Do you know if googlebots can still crawl the transcripts now that they're in the markdown blocks? Just wondering if I should also include the transcripts somewhere else on the site so the bots can find them. Thank you!
Hi there - I found your page and this code worked like a charm in my clients SquareSpace private page. We're trying to do accordion style drop downs for PDF's of reports for the year. The one thing I'm having trouble with though is when I create a link in the HTML 'Mark-Up' block it's linking to the PDF, but it doesn't show an underline and change the color of the text to indicate that it's a link. Thoughts? I guess worst case scenario I could always create PDF images that link to the document that say "Download" or something. Let me know what you think I can do. Wondering if the jQuery code just isn't taking the link CSS formatting from the SquareSpace template anymore.
I have got this working but can anyone tell me how to change the body font size? I want to be using my smallest which is Paragraph 3.
Title Text size
I tried accouple of things added #### for h4 visluallit it worked but however the accordion function does not work. All blocks are extended. I did try changing the script h2 to h4 but that did not work either
does anyone know how to use this accordion feature and change the title font size to h4
Hello,
It works for me, but if I try to do it on the section bellow it doesn't work.
Seems like I can't do it 2 times on the same page...
Do you have any tips ?
Thanks !
here is the test link https://begonia-rust-haew.squarespace.com/-switchingover-to-lithium
I have place the example text into a markdown box
placed the example script into the page/advanced
The result is the text show below, what am I missing
--------------------------------------
+ How does it work?
The solution uses a Markdown block...
The native Squarespace..
It supports bullet points and other styling codes
Markdown blocks can also include html...
jQuery is a...
Hi,
I am new to SQ and have a a need for a Accordion Tab to display a ton of FAQ's I am 100% lost as to where the example code is place. Which code goes where and the JX script?
Scott
Thank you Silvia! Do you know if this will work if it is placed inside a Product Info/Description box?
Have you been able to get anchor links working? I have many FAQs and would like to deep link to them. Thanks!
I'm testing this out on a free trial site. Will this code injection work if it's not a paid plan? I've tried inserting the markdown code and jQuery into the page's header code injection but it's not working.
Thank you heaps Silvia for this, I'm using @Mark's combined version successfully. It even works on mobile – almost.
There's a little snag with it for the answer to vertically centering, which means that for longer answers you always need to scroll back up to the top – can anyone help with this?
The site is https://www.thecarolamoon.com
Thank you, Carola
@Mark, your solution is perfect, it even works on mobile! YAY!
A few uni codes got into your code, here is the clean code that's working for me:
/*To add to site wide header injection:*/
<script type="text/javascript" src="//ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js"></script>
/*To add to page header injection:*/
<script>
$(document).ready(function() {
$('.markdown-block .sqs-block-content h3').addClass('ui-closed').css('cursor', 'pointer');
$('.markdown-block .sqs-block-content h3').nextUntil('h3').slideToggle();
$('.markdown-block .sqs-block-content h3').click(function() {
if ($(this).nextUntil('h3').is(':hidden')) {
$('.markdown-block .sqs-block-content h3').nextUntil('h3').slideUp();
$(".markdown-block .sqs-block-content h3").removeClass('ui-open');
$(".markdown-block .sqs-block-content h3").addClass('ui-closed');
$(this).nextUntil('h3').slideDown();
$(this).toggleClass('ui-closed ui-open');}
else
{
$(this).nextUntil('h4').slideUp();
$(this).toggleClass('ui-closed ui-open');}
});
});
</script>
Thanks for this! It fixed my issue - other than ONE error in the code:
$(this).nextUntil('h4').slideUp(); --- should be ---
$(this).nextUntil('h3').slideUp();
Once I fixed that, it worked perfectly!! So happy, as the code I was using from this page was not quite working properly and this is finally the fix I've been searching for. Thanks again!!
Hi, I know it's been a while, but I'm looking to make a "table of contents" for my FAQ that is just a list of links to the questions (using markdown anchor links). I'd like to click the link and go to the FAQ question and have it slide down to open it. Does anyone know how to make this work?
Thanks so much!
Hi Jack, I am looking for the same solution.
Any luck with this Jack/Alex? I'm looking to achieve the same thing?
I used variation 2 on my website and everything works perfectly but one 'bug' that I can't seem to get rid of. I can open one mark down box but it won't close if I press on it again. It seems to try to close and immediately reopens again. The only way to close it is by pressing on a different mark down block. Does anyone have this issue too?
This is the code I used:
<script src="//ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js"></script>
<script>
$(document).ready(function(){
$('.markdown-block .sqs-block-content h4').addClass('ui-closed').css('cursor','pointer');
$('.markdown-block .sqs-block-content h4').css('cursor','pointer');
$(".markdown-block .sqs-block-content h4").nextUntil("h4").slideToggle();
$(".markdown-block .sqs-block-content h4").click(function() {
$(".markdown-block .sqs-block-content h4").nextUntil("h4").slideUp();
$(".markdown-block .sqs-block-content h4").removeClass('ui-open');
$(".markdown-block .sqs-block-content h4").addClass('ui-closed');
$(this).nextUntil("h4").slideDown();
$(this).toggleClass('ui-closed ui-open');
});
});
</script>
I've read that you have to disable AJAX LOADING in the settings if you're using 7.0. Squarespace 7.1 does not have AJAX LOADING.
This too is something I would like to find a resolution on. One should be able to simply collapse the text back into the heading without having it jump back out.
I used the 'Variation 1' and it worked well. However, there is a random + showing up beside my third drop down that toggles whenever any drop down is clicked. Anyone know how to fix this?
Hi and thank you for the code! I've used 'Variation 2' on my Squarespace pages which has worked seamlessly.
I just went to use it as part of an Index Page and the +/- icon and dropdown functions stopped working -- any suggestions for me? Thank you in advance!
Yeah, it's glitchy. Only works when you refresh the page, not when you first navigate to it :(
Sorry! I disabled Ajax loading and it works like a charm! Definitely do so too if you're finding that it's not working.
I'm having the same issue. However, I don't have Ajax loading as a feature. Not sure how to resolve the issue?
Hi There, Thanks for the code! I'm looking for some assistance on the following;
Currently I'm looking to make a small addition to variation 1, where I can retract all content blocks (for example when you're finished reading the FAQ). Hoping someone can assist! I believe Ninjunc is onto the same - however i can't seem to get his code to work for me. He suggested the below, which i adapted to H4.
code below:
<script type="text/javascript" src="//ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js"></script>
<script>
$(document).ready(function(){
{
{
$(‘.markdown-block .sqs-block-content h4’).nextUntil(‘h4’).slideUp();
$(this).nextUntil(‘h4’).slideDown();
} else {
$(this).nextUntil(‘h4’).slideUp();
};
});
});
</script>
finally got it! Combination of all options; "manual open/close" & "toggle +/-" & "automatically close when another "H" is opened". For those interested:
<script type="text/javascript" src="//ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js"></script>
<script>
$(document).ready(function() {
$('.markdown-block .sqs-block-content h4').addClass('ui-closed').css('cursor', 'pointer');
$('.markdown-block .sqs-block-content h4').nextUntil('h4').slideToggle();
$('.markdown-block .sqs-block-content h4').click(function() {
if ($(this).nextUntil('h4').is(':hidden')) {
});
});
</script>
Hello, it woks great! How to set the first item to be open by default? Thanks
Hi there! I am using Variation 2 on my site and it's working like a charm, with one caveat: when the page loads, all the accordions are open instead of closed. :) Any idea what I could be doing wrong?
Mine is doing the same thing- they are all closed when I refresh the page. Did you figure it out?
nm, turned off Ajax loading and all is good.
Hey, thank you for this code. Can someone help me to change the size of the Heading? Or better, instead of using H2, use H1? I tried changing the "h2" in the script to "h1" but the code doesn't work.
Hi Max,
You would then also have to change the headings in the markdown block to h1! It should work.
Thank you very much for the code! Much appreciated :)
Amazing! thank you so much - I am a complete newbie but Variation 2 - Toggling the + / - symbols, is not working on my page.. how can I fix that?
I have inserted all the codes in the Code injection header section.. but the Variation 2 - Toggling the + / - symbols isn't working... who can help?
Thanks so much for this! Is there a way to have one toggle already open when the page loads?
Last week the code was working, but this week, its being super glitchy. I've looked thru here and notice others are having to refresh, but aside from that, now each text toggles 3-4x before expanding completely. It's crazy! Help!
@Morgan - were you able to get this glitch working? Having the same issue...
Hi - the code seems to be sort of working. When I first open the page the text in the markdown box is open/showing. When I hit refresh the text in the markdown box is hidden. Anyone have a suggestion for how to make the code hide when the page first opens?
Also, the same code is not working on the blog post page - even when I hit refresh the text does not 'hide'. Do you know if there is a reason this might not be working?
Maddie, I am having similar issues--did you get it figured out?
I found this site and the content is awesome. I'm not a Developer and attempting to create a site via Squarespace. My question for all, and please don't kill me, am I to insert the code for the Markdown and I assume I add my content within the code, correct? Any help is not only Appreciated but very much Thanked.
Thank you for this! As a newbie, I wasn't sure where to add the custom CSS code (don't laugh). I finally put it in the page injection code after the script, but it needed a a <script> tag. For other newbies, it should look like this:
<script>
.markdown-block p {
margin-left:1.5em;
}
.markdown-block .ui-closed:before {
font-family:monospace;
content:"+ ";
}
.markdown-block .ui-open:before {
font-family:monospace;
content:"- ";
}
</script>
Thank you so much for this! Amazing!! Is there a way to have the first question expanded ? I can't figure that out! Thank you!!
Hello, I'm using the Brine template for my site! I have an index that contains several pages. Some pages are just banner images with a title, while the others pages have the accordion drop down menus from this tutorial. The accordian menus work as intended except for the fact that they mess up the rest of the index by making the banner images invisible.
I thought it was something else and I was checking site styles, but once I got rid of the script in the code injection for the index all of the banner images started working again! But then obviously the accordian menus don't close.
Any ideas on why this is happening?
There's a fix on squarespace answers that worked for me. See Zahra's answer: https://answers.squarespace.com/questions/232935/accordion-dropdown-only-working-on-refresh-even-if.html?childToView=273844#answer-273844
I'm currently using this code on an index page of squarespace. I'm noticing that the sections bleed into each other when I open a markdown. Has anybody else run into this? And if so, were you able to fix it?
Hello! Thanks for the tutorial! I've managed to get it to work for a regular page, but when I use it within a gallery page, it is displayed as expanded and doesn't collapse when clicked. Anyone has any idea why that is? I'm using the Montauk theme.
Hello! Thanks for the tutorial, it is working great—exactly what I needed for my website. One question though, for the hyperlinks, is it possible to have an option to "open in new window"? Thanks!
Has anyone figured out how to get this to load properly the first time around? Mine only shows up correctly on refresh
Hi Briana,
Make sure you have Ajax loading turned off in the site styles!
I've implemented this code as directed above however the functionality doesn't activate until the page itself is refreshed then everything works fine. How do I get around that so the upon initial load of the page the functionality activates and not having to refresh? here's the page itself https://www.roosthomewatch.com/faq .
Can someone please answer this quesiton? I am struggled by this too. Even in this page, if you first enter it, the text are expanded when first loading and collapsed once the page is fully loaded. Anyway that the expanded texts are collapsed at first?
Is there an updated jQuery code for the markdown text? I added it but nothing seemed to change and the drop-downs are not hidden. Thanks.
I know this is an old post. But it is so good I was using it again in a new project. In case anyone could use this, I threw in an "if/else" statement to combine parts of Variation 1 and Variation 2 (toggle and auto-collapse when new FAQ is clicked). Here is what worked for me (note, I am also using h3 for my implementation):
Hi Ninjunc. Am just teaching myself a bit of Markdown for my website. I have the original script running perfectly (Variation 2; +/- toggling) but would love to add your bit so that the displayed answer retracts when a new FAQ is clicked. Should I be substituting your code for the original or adding it underneath and which parts of my text should I be making h3? Kind regards, Charlie
I am using the Five template, and the styling looks perfect, but the scripts are not working. Can someone please help?
Do you know if it is possible to centralise the text?
Can I do two hide / show levels?
For example, open h2 and inside h2, open h3?
Im trying that:
<script>
$(document).ready(function(){
$('.markdown-block .sqs-block-content h2').addClass('ui-closed').css('cursor','pointer');
$(".markdown-block .sqs-block-content h2").nextUntil("h2").slideToggle();
$(".markdown-block .sqs-block-content h2").click(function() {
Thank you for providing this code! However I do have a question about an unwanted side-effect…: After having done this, a lot of my other blogposts and pages suddenly got some paragraphs with random indents… Rather annoying, and I do not wish to go into all previous blogposts and try to change this in all. Do you understand why it happened? (See f.ex point 2, 3 and 5 here: https://superstov.com/blog/2016/5/5/three-things-i-didnt-know-about-research)
Nevermind this question! I found out myself when I just used my brain to think a little… :D
Hi there,
Do you know if this works on the Pacific template?
Thanks
Hi there :)
I have followed all the steps and have used the updated code I saw in the comments when it wasn't working. But it is still not working :/
See my below coding used:
Settings > Advanced > Code Injection:
<script type=“text/javascript” src=“//ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js”></script>
Page Settings:
<script>
$(document).ready(function(){
$('.sqs-block-code .sqs-block-content h3’).css('cursor','pointer');
$(".sqs-block-code .sqs-block-content h3”).nextUntil("h3”).slideToggle();
$(".sqs-block-code .sqs-block-content h3”).click(function() {
$(this).nextUntil("h3”).slideToggle();
});
});
</script>
Design > Custom CSS:
.markdown-block p {
margin-left:1.5em;
}
.markdown-block .ui-closed:before {
font-family:monospace;
content:"+ ";
}
.markdown-block .ui-open:before {
font-family:monospace;
content:"- ";
}
Please help
Hi, thank you for this really useful code. I have a question:
each time you refresh the page, the hide/show section is expanded by default and then it shrinks instantly, is there a way to prevent this effect? It's no big deal, but it makes the page look a bit unpolished
Hello! my English is not good. I do not know anything about CSS but I was reading a bit and I think I added some things like borders, color and centering it (I do not think it's a big deal for experts, but it made my day). What I have not managed to do is open Ask, and close it. If I make the attempt to open and close, it opens again.
// The script
<script>
$(document).ready(function(){
$('.markdown-block .sqs-block-content h3').addClass('ui-closed').css('cursor','pointer');
$(".markdown-block .sqs-block-content h3").nextUntil("h3").slideToggle();
$(".markdown-block .sqs-block-content h3").click(function() {
$(".markdown-block .sqs-block-content h3").nextUntil("h3").slideUp();
$(this).nextUntil("h3").slideDown();
$(this).toggleClass('ui-closed ui-open');
});
});
</script>
//CSS
.sqs-block-markdown h3 {
border: solid 1px #D3D3D3;
padding: 20px 14px;
outline: #D3D3D3 solid 2px;
outline-offset: 5px;
}
.markdown-block p {
margin-left:1.5em;
}
.markdown-block .ui-closed:before {
font-family:monospace;
content:"+ ";
}
.markdown-block .ui-open:before {
font-family:monospace;
content:"- ";
}
Thank you for this! Could you tell me what I'm possibly doing wrong with the code? When I'm logged into my site and editing the page it looks great and works, but when I go to the actual web page in another browser all of the questions are expanded. Perhaps you can help? https://heiprodigital.com/faq
I'm having the same issue with my site. Can you help?
Here's the page: https://www.groveemotionalhealth.com/collaborators/
Thank you!
I figured it out! If your text is initally loading expanded (but contracts when you refresh) go to your style editor and disable ajax loading.
Thank you Carryn! That did the trick! Bummer about the rest of the site not being able to have ajax loading though, I wonder if there's specific CSS code that would work just for the page with the hide/show functionality.
This doesn't seem to work for Mobile versions of the website, despite working on the desktop.
Thank you for this. It worked beautifully for me. Only question I have is if there is a way to have links open in a new window? I tried inserting html code but that doesn't work.
i use the pacific template and sadly it doesn't work. i Tried the posted code as well as the suggested version bei Shae D., none of it worked not matter where i injected it.
Any idea? I use the pacific template.
You are awesome for sharing this! Thank you so much!
Great code Colin! Works great on my site. I was wondering though is how would I alter your Jquery to change the text in the Markdown block that triggers the accordion dropdown. For example, on my site (https://tito-chazo-pakj.squarespace.com/clients/) if you scroll down to the "Customer Success Stories" you will see the Markdown block. Clicking on the "Read More . . . " link triggers the dropdown. Using Jquery, how do I change the "Read More . . ." text to "Close" after being triggered? Thanks!
Hi! I am trying to find out the same thing, and see that you found out how to do this on your website. Could you tell me how you changed this?
Did you manage to figure this out? Having the same problem with my site. Thank you so much.
This code no longer works on some Squarespace templates as the CSS class has changed for the code block. Use the below for Step #2 instead. Also if you are using an "index" page layout, the code must be installed in the code injection for the index page itself.
<script>
$(document).ready(function(){
$('.sqs-block-code .sqs-block-content h2').css('cursor','pointer');
$(".sqs-block-code .sqs-block-content h2").nextUntil("h2").slideToggle();
$(".sqs-block-code .sqs-block-content h2").click(function() {$(this).nextUntil("h2").slideToggle();});
});
</script>
Do you know which templates it doesn't work on?
Firstly , thank you for the code , it has helped me to improve a big issue on a site im building .
I have one problem though , the code dosent seem to work when using Safari ? Is there a way to fix this ?
Above example does not work with Safari either ..
Hi,
I installed the script and it basically works, but I ran into a few of small problems. First, after the page loads initially, the questions were all open and couldn't be closed. You have to refresh the page to get it to work right. Turning off the Ajax loads got this to work the way it should.
Second, when a question is clicked to show the answer, the entire page jerks left a few pixels, and then jerks back when you close the question.
Third, when the page first loads the questions are open until, it seems, the custom code loads and executes. Is there some way to specify in the markdown block that these questions have display:none to begin with, so they don't display until the custom code loads and does it's thing?
Thanks for the code.
Hi Steve,
I had the exact same problem. How did you turn off Ajax and could that negatively affect other aspects of the way the website functions?
Thank you!!
Hi, great article. How would I center the text and + symbol instead of having it left justified?
Hi! I followed the instructions but the function doesn't works. Could you help me? Thank's in advance
Thank you so much!
Great script..
Is it possible to link to a certain tap using anchor?
Right now i have a link that links to #FAQ on my about page - but i would like to be able to specifically target one of the tabs/accordions, so when you arrive on the page, that one tab/answer is open
Hi! I have the same problem! I want my first question to be open. Any ideas?
Thank you so much. This worked beautifully.
This is excellent and worked a treat. Thanks heaps.
Hi,
I'd love to know if there is a way to implement this same idea, but for read more/show less buttons? So far, I am able to expand text, but the read more button remains visible in the middle of the section where I am expanding text.
Thanks in advance,
D
Hi,
I'd love to know if there is a way to implement this same idea, but for read more/show less buttons? So far, I am able to expand text, but the read more button remains visible in the middle of the section where I am expanding text.
Thanks in advance,
D
Hello, I added the code for this and there seems to be a small issue. If I click on the FAQ page https://www.mabuhaywebsites.com/faq/ it comes up without the accordion....but if I refresh the page it works fine. Any idea why thats happening? Thanks in advance! and thanks for the code!
Im having the same issue :(
I'm having the same issue too, where you ever able to fix that?
You're a LIFESAVER. Thank you so much! I had no idea how to do this but it works perfectly.
Hi Colin,
Thank you so much for this code. Its working perfectly. Im using this not for FAQs but rather Services offered and its description. I do have 2 queries
1. I'm using the variation 2 in which if you click some other "service" the other collapses. Can I make the same tab collapse? Right now if I expand one option it doesn't retract by clicking it again. I hope you know what im saying.
2. Is there a way I can use regular font instead of Headings? I played around a little and tried H3 too but isn't what I really want.
Can you help please ?
Thanks a lot .
Deep
Variation 1**
Ok so for all the folks experiencing the same problem that I had. If you have your Hide/Show content already expanded when you enter your page but collapsed as normal when you refresh:
It is because you have the code duplicated in your section while it should only be in your top index/section (the master one). Also you should probably disable Ajax loading I guess... I don't know really.
This is the problem I'm having! Accordion is loading already expanded. I have two sites set up through square space, using the exact same template....works on one and not the other. I can not figure out what I'm doing differently! I'm adding a Markdown block within the page content and adding it there. Am I doing that wrong? It worked on the first site.
Thanks! This saved me heaps of tearing hair out!
I am looking to incorporate both Variation 1 and Variation 2. I can get either to work on their own, but can't figure out how to get the functionality of both variations to work.
Anyone have any ideas?
You are amazing. For the life of me I haven't figured out why SquareSpace doesn't have this functionality built-in but people like you really make the world work a bit better. Thank you.
This is to expand on my comment below: I messed around a bunch with the code. I left the first jquery src code in the page injection, then took the other two parts out and put them in a code block in the top of my page and the banner issue went away and the markdown hide/ show content still works.
Now I have the same part of the code showing up on my page.
This is the code that was showing up on top of my banner image and now shows up on my page:
.markdown-block p { margin-left:1.5em; } .markdown-block .ui-closed:before { font-family:monospace; content:"+ "; } .markdown-block .ui-open:before { font-family:monospace; content:"- "; }
Is there an adjustment to the code that I should make so it stays hidden?
Thanks so much!
you need to put this css back in your code header injection point. Then once you do that, you need to put it within the <style> tags. It will look like this
<style>
.markdown-block p {
margin-left:1.5em;
}
.markdown-block .ui-closed:before {
font-family:monospace;
content:"+ ";
}
.markdown-block .ui-open:before {
font-family:monospace;
content:"- ";
}
</style>
You should wrap the css part in style tags: <style> ..the css.. </style>
mine works but has two + symbols. one changes from + to minus. the other just sits next to the word and does nothing. how can i remove the one that doesn't change?
The + is automatically added via the CSS. I am assuming you are adding a + to your question. If so, remove the + from your question in the markdown.
Hi and thanks so much for this amazing piece of work. It finally helped after months of searching!
Had one issue I was hoping you might be able to give me some advice on: I entered the code into my page header in Squarespace. Then I created a markdown block and made my hide/ show content. (I used the manual hide/ show variation #2)
Everything is great except I now have a black strip running across my banner image and if I highlight it I can read the page header code in the black space.
Do you know what might have happened or how I could fix this? My page address is: https://www.freshadventures.ca/vancouverislandtraveldeal
Thanks so much for any help you can offer!
How can I make this horizontal rather than vertical?
Having problems adding this to my site. The list is expanded and won't collapse. I entered the JQuery in header code injection and the clean script in the page injection. I added CSS in custom CSS. Tried putting jQuery in page injection instead, but still no luck.
i've also disabled Ajax loading in the design style editor
I got the same problem as you do. Anyone has a solution ?
But it does come back to a normal (collapsed) form when I refresh the page...
Just a problem that I've run into regarding this. Well actually two.
I have a paralax image that comes after this block and it will not show until I expand the text. It seems to be compensating for the full length once the text block is revealed. The other way that the image eventually reveals itself is by resizing the window, so it kind of refreshes itself. Odd.
Also the second issue is that when I go to another location on my site then come back to the page with the expandable text blocks they no longer have the expandable capability, and all of the concealed text is actually visible! Like there wasn't even the concealment to begin with. Do you know why this might be the case?
For the first problem there is no easy fix. Parallax works by calculating all sorts of content heights and then positioning background images and applying transformations/animations to them to create the parallax effect.
For the second problem I think you probably need to disable Ajax loading in the Style Editor.
Is there any way to have the first ajax answer pre- opened on load?
Thank you so much for your post, i'ts much appreciated. Worked first time.
Tom
AH! THANK YOU SO MUCH!!!! If you can't already tell, I'm stoked I stumbled across your page on Pinterest. Thank you a million times over!
Hello, is there a way to treat the + or - as a bullet (i.e. indent any following text in future lines to the start of text after the+ or -)
I just wanted to leave a quick not, this is so unbelievably helpful and such a fantastic bit of code. It totally took my project to the next level.
High fives, Andy
This didn't work for me. I injected the first script piece of code into the site-wide code injection space. then I went to the advanced settings of the specific page i wanted the markdown in. I pasted the script and the css code for "Variation 2". It doesn't appear to be reading the css code after the script code because the font isn't changing colors to recognize the names of things as code usually does. Am I supposed to be posting code in 2 different languages next to each other, or am I not pasting them in the right place? I then added a markdown block and pasted the code for the markdown above just to test. It doesn't work, it just shows all of the text. Please help!
Colin you're a sorcerer... thank you so much!! I got everything working fine. But much to my surprise, when I tried testing on Chrome, all the Qs & As loaded collapsed followed by a 0.5s transition where the jQuery toggled them close. The page loads fine on Safari. Is there a fix in the script for this? Thanks again... you're amazing!!
Hello, thanks so much for sharing this. Can you please explain how (where) to add the custom CSS? I see there is a way to enable Developer Mode, which I believe gives me access to CSS editing (or CMS? sorry I don't know the correct reference) but I am a little hesitant. I would prefer not to have to SFTP or use Git... would rather do it all from my web browser as I am not a software developer. Thanks again. ~N
OK... I posted too soon, sorry. I figured out how to add custom CSS (under the Design menu). But now a problem ... Instead of one "+" beside each Header question, there are two columns of "+" symbols. The first column toggles (added by the CSS). The second column does not (text in the Markdown field). It looks a little silly. Not the worst thing, but I would prefer to fix. Advice? Here is my URL: https://judaicmosaic.org/faq/
Go to the code you put into the Markdown block, and remove the + before the question.
thanks!
Quick question, does anyone know if this code works on
Safari and iOS10? I used some code to get this effect from another site and it works fine except on Safari and iOS10. So, will need to replace the code i'm currently using, but don't want to imput new code if I'll have the same problem - any advice super appreciated
View this page using Safar / iOS10 and see if the examples at the top of the page work.
any way of combining both variations? the toggling of +/- and make the answer retract when another is opened?
i tried playing around with the code, but javascript is out of my league still
Try this:
<script>
$(document).ready(function(){
$('.markdown-block .sqs-block-content h3').addClass('ui-closed').css('cursor','pointer');
$(".markdown-block .sqs-block-content h3").nextUntil("h3").slideToggle();
$(".markdown-block .sqs-block-content h3").click(function() {
$(".markdown-block .sqs-block-content h3").nextUntil("h3").slideUp();
$(this).nextUntil("h3").slideDown();
$(this).toggleClass('ui-closed ui-open');
});
});
</script>
Is there a way to implement this using the normal text style instead of the heading text? I have it working on my page, but my theme only supports one style of header (not h1, h2, and h3), so the text is showing up huge. If I edit it in style editor, it ruins all the headings on my site.
Thanks so much for this very useable, useful post!
I'm using variation 2 where it toggles between "+" and "-".
Is there a way to use the "^" and down arrows in place of the "+" and "-"?
How would I do that?
Change the CSS to the following.
.markdown-block p {
margin-left:1.5em;
}
.markdown-block .ui-closed:before {
font-family:monospace;
content:"▲ ";
}
.markdown-block .ui-open:before {
font-family:monospace;
content:"▼ ";
}
I am having the same problem with the + with the FAQ and the answer below, it won't toggle off. Here is a link to my page:
https://www.aspenepic.org/income-volatility-survey-results-1
My Markdown block is showing the + with the FAQ and the answer below, but is not retracting the answer. Where do I need to put the above coding to able to toggle on the site using the + before the FAQ?
Can you post a link to the page you have the markdown block on?
Is there any way of setting "section" headings using this method? The Script looks for the next H2 tags and uses that to know when to close an answer. When I drop "section" headings in, they get wrapped in the answer that is above it:
Section Heading 1
Question 1
Answer...
Section Heading 2 (gets sucked up into the answer from Question 1)
Question 2
Answer...
To use the jquery code on github for instance, how should I rename the .sqs-block-content?
Tried to use the code as provided but didn't work.
Hey Colin.
I know a few people have already asked but I couldn't find an answer.
Whenever I punch in the code in the appropriate areas, I simply get the questions with the text answer right beneath them with no ability to expand and retract.
Please advise!
Edit - I put in the code in the Index instead of the page and the function works now BUT whenever I click the question it simply retracts than automatically expands by itself. The answer section just pops back out after it hides for a moment.
Can you get in touch via my contact form? Be sure to include a link to your site.
Thanks so much for providing this, Colin! I'm having a small problem, though. Not sure what might be the issue with it.
The first time I load the page, the show/hide function doesn't work. But, when I force a page reload, it works. Any insight into what might be the problem?
Here's the page in question: https://www.azps.life/our-story/
Interesting, when I copy/paste the address directly, it works every time. If I use the link in my navigation along the top of the page, though, it presents with the problem I described above.
Read through comments to see if someone else already solved this, but didnt find anything...Is there a way to force selective links in a Markdown box to open in a new window? The option isn't appearing, but this client's site has several links, which I'd very much rather have open separately. Thank you!
You can use standard html links in Markdown, so an <a href=... with target = _top would do the trick
I think I understand. I inserted them as hyperlinks using that function in Markdown. But the problem is still present. This is the site: https://uptownconcierge.squarespace.com/faq
What do you mean by html and the <ahref=...?
My Markdown looks like this for example:
es! We offer a FREE 30 minute consultation that you can book online - BOOK NOW
Ahh I figured it out what you mean now. Got it working! Thanks!
Hi AC,
Could you please explain what you did here? i've pasted the html into the Index page and html into the actual page with the Markdown content.
It doesn't collapse until I refresh the page.
Desperate to find out how I can make this work. i'd really appreciate your advice. I'm not a programmer so code to copy and past would be very much appreciated.
Many thanks,
Anna
Hi Colin.
First, thank you for this post and the code. You are an absolute legend!
Second, I'm unsure how you would use the html links you suggested above for opening hyperlinks in another window. Could you give me an example of how to use this, Colin or AC?
Thank you :)
This isn't working on my site. I put the jquery code in settings/advanced/code injection/header and then put the clean version code in design/custom css
what am i doing wrong?
Thank you, this is really nice and simple. I may have missed it in the comments, but is there an option to combine Variations 1 and 2? Toggling and retraction?